Step 1: First, we will design a simple button using HTML. Refer to the comments in the code.
- index.html
<h1>Increment and Decrement counter</h1>
<div="container">
<!-- adding button and heading to show the digits -->
<!--increment() and decrement() functions on button click-->
<button onclick="increment()">+</button>
<h2 id="counting"></h2>
<button onclick="decrement()">-</button>
</div>
Step 2: Next, we will use some CSS properties to design the button and use the hover class to get the animation effect when we hover the mouse over the button.
- style.css
/apply css properties to body tag/
body {
position: absolute;
left: 0%;
text-align: center;
}
/apply css properties to container class/
.container {
justify-content: center;
align-items: center;
display: flex;
height: 100%;
text-align: center;
}
/apply css properties to button tag/
button {
width: 90px;
height: 60px;
font-size: 30px;
background-color: green;
color: honeydew;
}
/apply hover effect to button tag/
button:hover {
background-color: greenyellow;
color: grey;
}
/apply css properties to h2 tag/
h2 {
color: black;
margin: 0 50px;
font-size: 45px;
}
/apply css properties to h1 tag/
h1 {
font-size: 35px;
color: green;
text-align: center;
padding-left: 10%;
}
Step 3: Now, we will add some JavaScript code to add functionality to the buttons which we have created earlier. Refer to the comments in the code for help.
- index.js
//initialising a variable name data
var data = 0;
//printing default value of data that is 0 in h2 tag
document.getElementById(“counting”).innerText = data;
//creation of increment function
function increment() {
data = data + 1;
document.getElementById(“counting”).innerText = data;
}
//creation of decrement function
function decrement() {
data = data – 1;
document.getElementById(“counting”).innerText = data;
}
Complete Code: In this section, we will combine the above three sections to create a counter.
- HTML
<!DOCTYPE html>
<html>
<head>
<!-- CSS code-->
<style>
/*apply css properties to body tag*/
body {
position: absolute;
left: 0%;
text-align: center;
}
/*apply css properties to container class*/
.container {
justify-content: center;
align-items: center;
display: flex;
height: 100%;
text-align: center;
}
/*apply css properties to button tag*/
button {
width: 90px;
height: 60px;
font-size: 30px;
background-color: green;
color: honeydew;
}
/*apply hover effect to button tag*/
button:hover {
background-color: greenyellow;
color: grey;
}
/*apply css properties to h2 tag*/
h2 {
color: black;
margin: 0 50px;
font-size: 45px;
}
/*apply css properties to h1 tag*/
h1 {
font-size: 35px;
color: green;
text-align: center;
padding-left: 10%;
}
</style>
</head>
<body>
<!-- give a suitable heading using h1 tag-->
<h1>Increment and Decrement counter</h1>
<div ="container">
<!-- adding button and heading to show the digits -->
<!-- increment() and decrement() functions on button click-->
<button onclick="increment()">+</button>
<h2 id="counting"></h2>
<button onclick="decrement()">-</button>
</div>
<!-- JavaScript code-->
<script>
//initialising a variable name data
var data = 0;
//printing default value of data that is 0 in h2 tag
document.getElementById("counting").innerText = data;
//creation of increment function
function increment() {
data = data + 1;
document.getElementById("counting").innerText = data;
}
//creation of decrement function
function decrement() {
data = data - 1;
document.getElementById("counting").innerText = data;
}
</script>
</body>
</html>
Output:
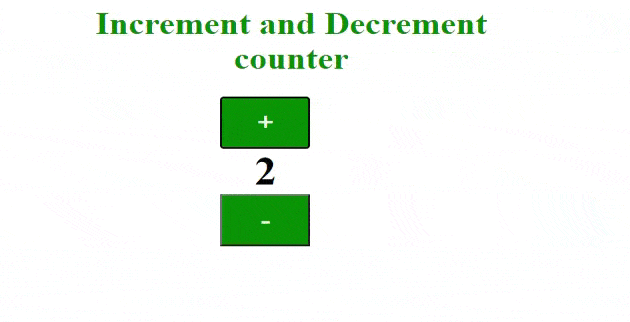